6.2.2 - SUI Containers
What's a container?
It's like a child window of a panel. In SUI it's declared as SContainer.
We can add one to a panel in mainly two ways:
- using the
-
sign - using .Add
// adding a container to the panel using the "-" sign (note that there is no semicolon at the end of the panel creation line)
var panel = RegisterNewPanel("panel id", true).Anchor(AnchorType.MiddleCenter).Background(Color.blue, EBackground.RoundedStandard).Size(1280, 720)
- SContainer; // adding the container to the panel using "-"
// adding a container to the panel using .Add
var panel = RegisterNewPanel("panel id", true).Anchor(AnchorType.MiddleCenter).Background(Color.blue, EBackground.RoundedStandard).Size(1280, 720);
panel.Add(SContainer); // adding the container to the panel using .Add
Working with containers
From my experience containers behave similarly to panels.
We can change their size, position, AnchorType, style etc. just like panels.
After adding a container to a panel and giving it a green color we get the image below
var panel = RegisterNewPanel("panel id", true).Anchor(AnchorType.MiddleCenter).Background(Color.blue, EBackground.RoundedStandard).Size(1280, 720); // creating a blue panel
var container = SContainer.Background(Color.green); // creating a green container
panel.Add(container); // adding the green container to the blue panel
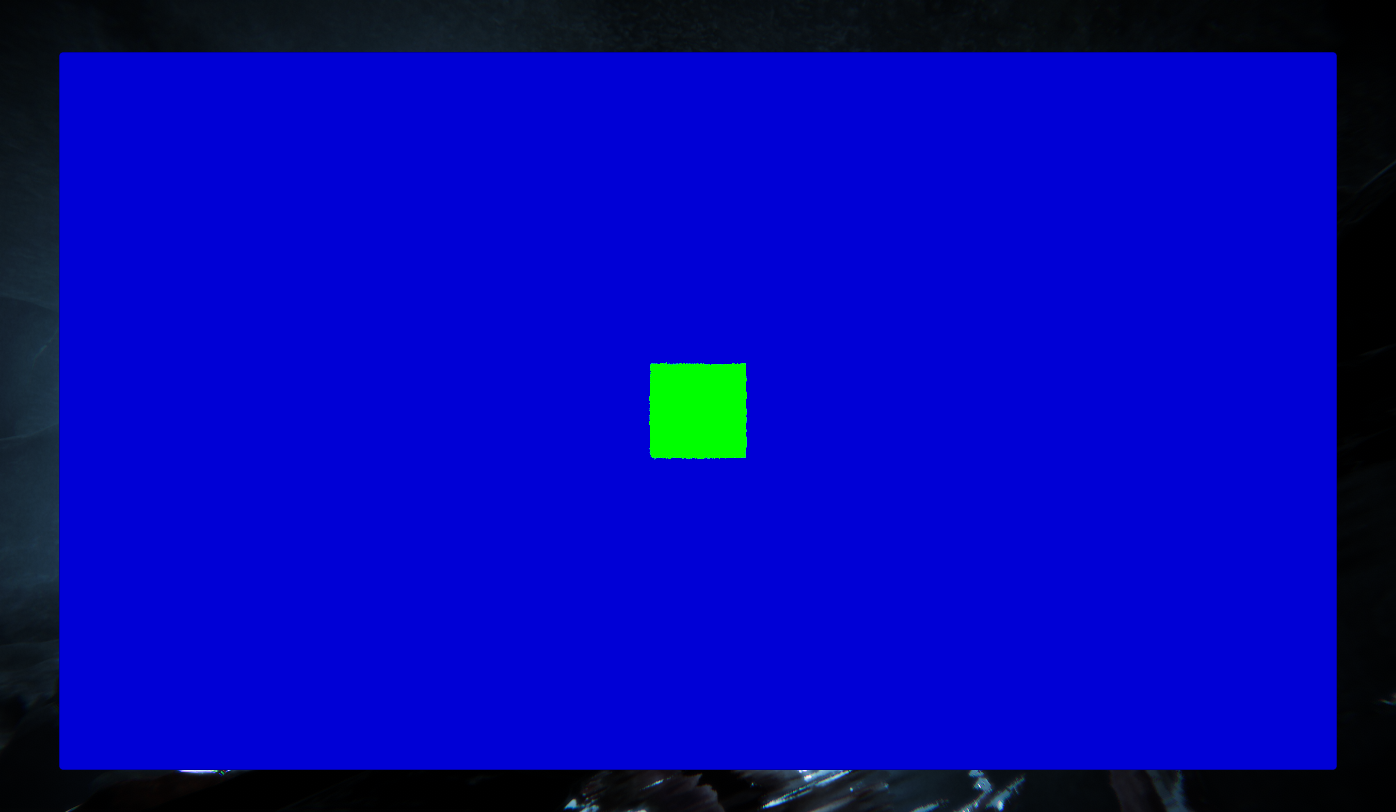
We can for example make it fill the panel by using the .Anchor property with AnchorType.Fill as value.
The Vertical and Horizontal properties
.Vertical and .Horizontal are used on a container to define the layout of it's child elements.
When the container is set to Horizontal it's child elements will be placed horizontally from left to right into the container.
// Creating an horizontal container with three labels in it
var panel = RegisterNewPanel("panel id", true).Anchor(AnchorType.MiddleCenter).Background(Color.blue, EBackground.RoundedStandard).Size(1280, 720); // creating the panel
var container = SContainer.Background(Color.green).Horizontal() // creating the container and declaring it's layout as horizontal
- SLabel.Text("1").FontColor(Color.black) // adding first label to the container
- SLabel.Text("2").FontColor(Color.black) // adding second label to the container
- SLabel.Text("3").FontColor(Color.black); // adding third label to the container
panel.Add(container); // adding the container to the panel
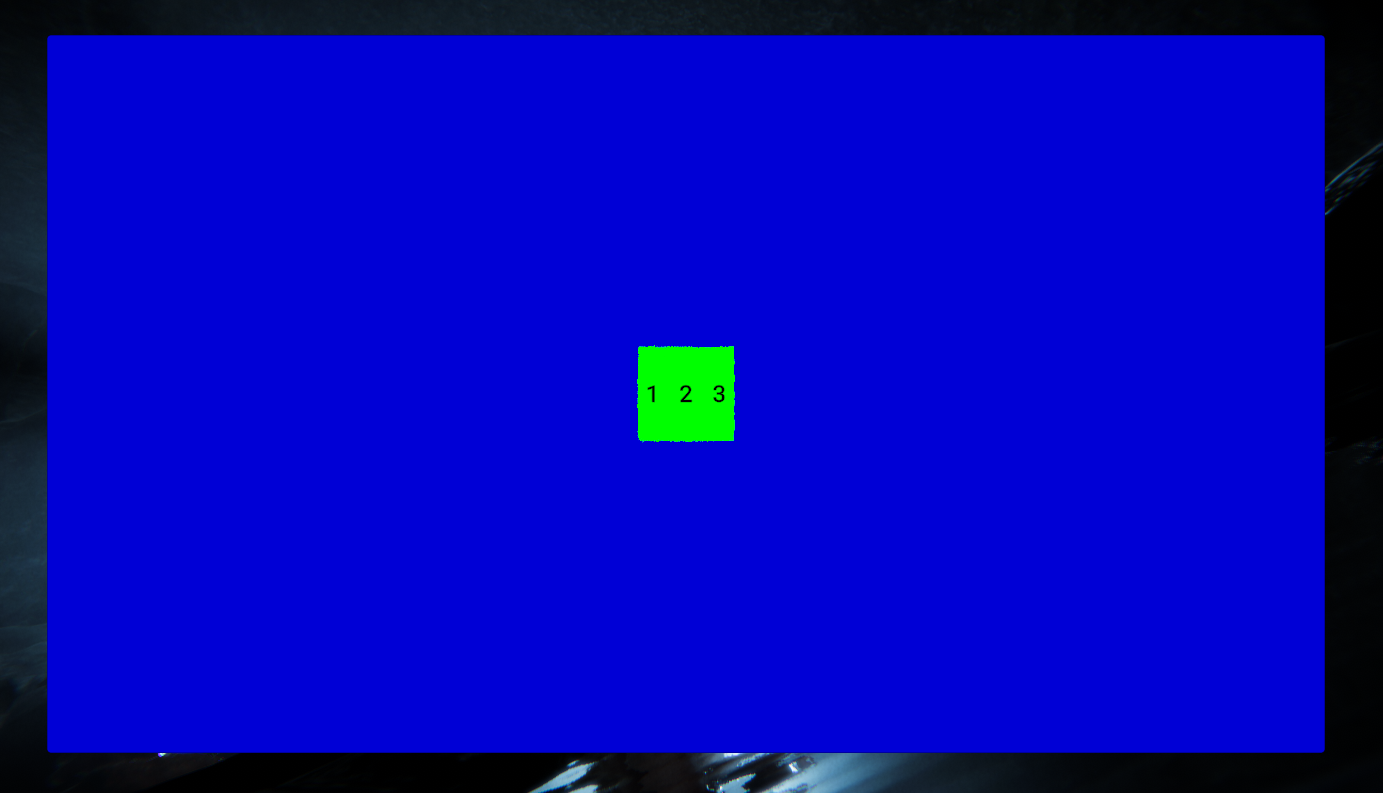
When the container is set to Vertical it's child elements will be placed vertically from top to bottom into the container.
// Creating a vertical container with three labels in it
var panel = RegisterNewPanel("panel id", true).Anchor(AnchorType.MiddleCenter).Background(Color.blue, EBackground.RoundedStandard).Size(1280, 720); // creating the panel
var container = SContainer.Background(Color.green).Vertical() // creating the container and declaring it's layout as vertical
- SLabel.Text("1").FontColor(Color.black) // adding first label to the container
- SLabel.Text("2").FontColor(Color.black) // adding second label to the container
- SLabel.Text("3").FontColor(Color.black); // adding third label to the container
panel.Add(container); // adding the container to the panel
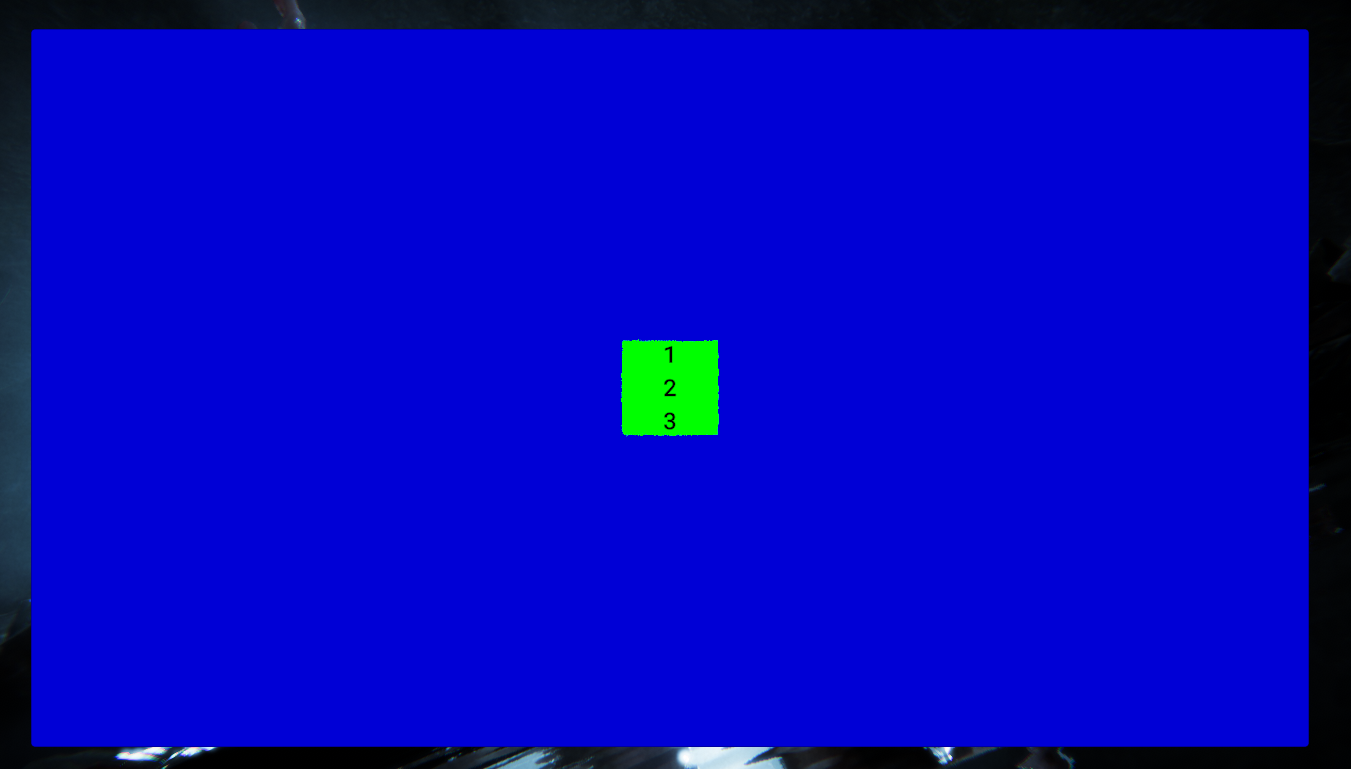
The VFill and HFill properties
We can use VFill() or HFill() on a container to either make it fill the panel vertically or horizontally
// Creating a vertical container which fills the panel vertically and with three labels in it
var panel = RegisterNewPanel("panel id", true).Anchor(AnchorType.MiddleCenter).Background(Color.blue, EBackground.RoundedStandard).Size(1280, 720);
var container = SContainer.Background(Color.green).Vertical().VFill() // creating the container with vertical layout and making it fill the panel vertically usin VFill()
- SLabel.Text("1").FontColor(Color.black)
- SLabel.Text("2").FontColor(Color.black)
- SLabel.Text("3").FontColor(Color.black);
panel.Add(container);
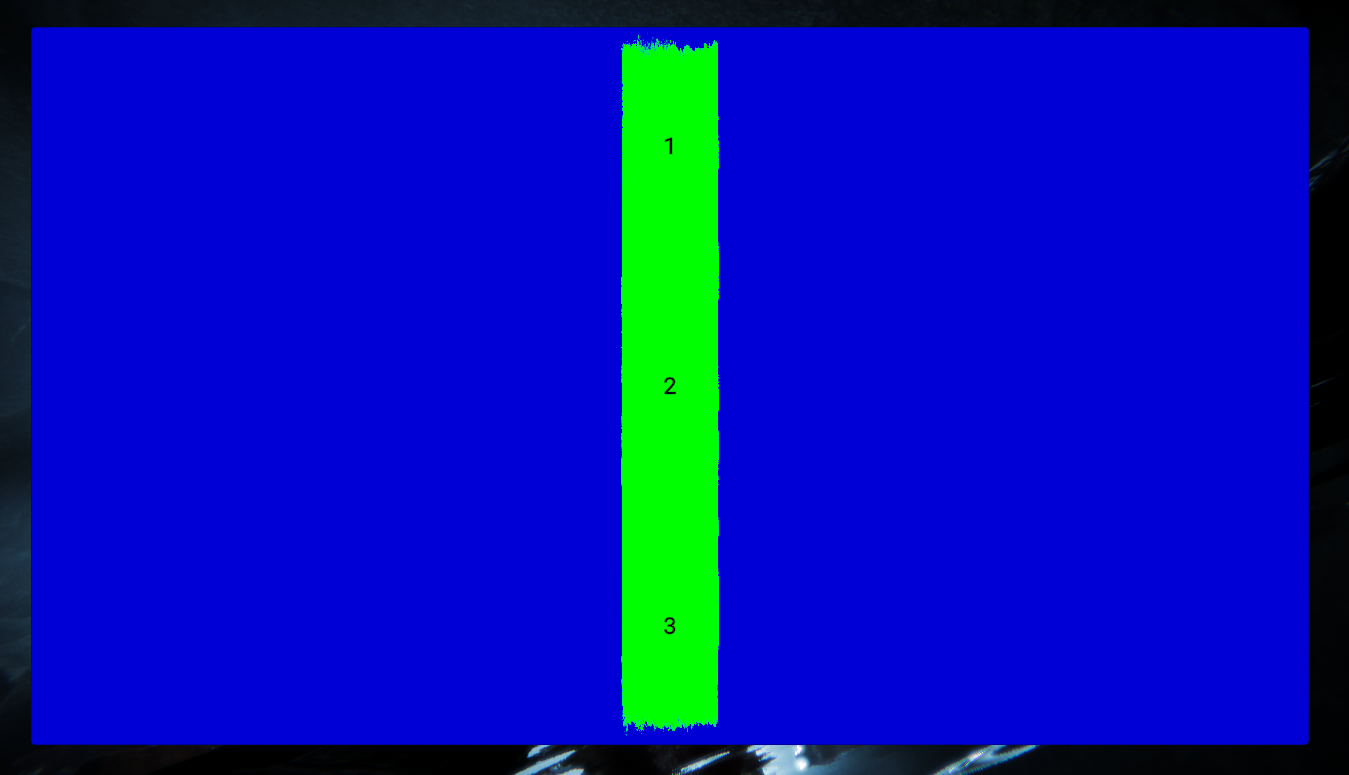
The same goes horizontally using HFill()